Introduction
By default, the way the EH-MC17 bluetooth module works is by echoing any data received through the BLE interface to the host device connected by tx and rx in hardware, or vice-versa. In the case of the 3DoT board, this means that any data sent from the 3DoT board’s ATmega to the bluetooth module will be repeated to any devices connected by bluetooth or vice-versa.
Details on this are found in section 1.1 of the EH-MC17 “Command Interface User Guide“, where all the information in this blog post is derived from.
Besides this default operation, sending commands with a certain syntax from the host device to the EH-MC17 can be used to check it’s status and change settings.
You can use the following Arduino sketch to communicate with the bluetooth module:
UARTcomm.ino
#define BAUD 9600 char c = ' '; void setup() { Serial.begin(BAUD); while(!Serial); Serial.print("Sketch: "); Serial.println(__FILE__); Serial.print("Uploaded: "); Serial.println(__DATE__); Serial.println(" "); Serial1.begin(BAUD); Serial.print("BTserial started at "); Serial.println(BAUD); } void loop() { // Read from the Bluetooth module and send to the Arduino Serial Monitor if (Serial1.available()) { c = Serial1.read(); Serial.write(c); } // Read from the Serial Monitor and send to the Bluetooth module if (Serial.available()) { c = Serial.read(); Serial1.write(c); Serial.write(c); } }
To test the sketch, upload it to your 3DoT board and open the serial monitor in the Arduino IDE.
Set the first dropdown at the bottom of the serial monitor to “Both NL & CR”
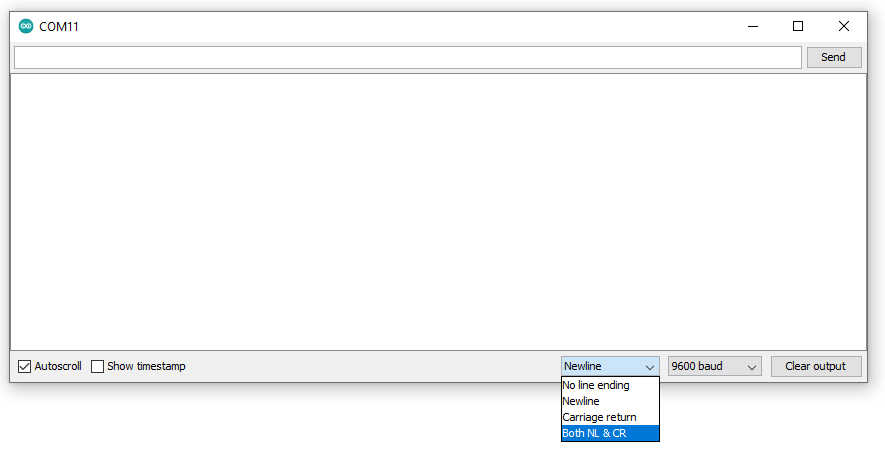
Try sending “AT”
If everything is set up correctly, the bluetooth module should respond with “OK”.
If you just purchased an EH-MC17 and soldered it to your 3DoT, you will likely not see an “OK” as the buad rate is set to 115200 by default, and we are using 9600 in our sketch. The accompanying 3DoT apps also use 9600 as the baud rate, so follow the instructions below to set this as the default rate.
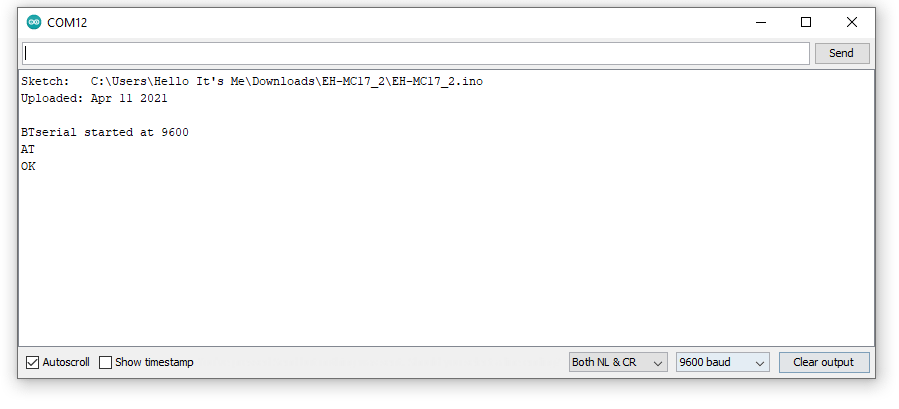
On the first line of your sketch, change
#define BAUD 9600 to #define BAUD 115200
Upload the code and open the serial monitor again. Send
AT+BR=9600
You may see some jumbled text returned – this is fine. (On some older bluetooth modules, you may need to use “AT+BAUD=9600” instead.)
Set
#define BAUD 115200 back to #define BAUD 9600
and upload the code once again. When you now open the serial monitor, AT should return OK. You are now ready to use your bluetooth-enabled 3DoT board, and the rest of the AT commands listed in the “Command Interface User Guide” should also work. For example, if you want to query the bluetooth module’s name that appears when searching for devices on your phone, send
AT+NM
If you want to change the name, send something like
AT+NM=My3DoT
You should see the bluetooth module respond with
+NAME=My3DoT
OK